Taking Your Java Skills to the Next Level
So, welcome to the next stage of your journey to learn Java! We’re now.. you’ve got the basics down. Now we delve into intermediate Java concepts that will allow you to write more efficient, scalable, and maintainable code. We’ll cover important aspects such as OOP concepts, exception handling, collections framework, generics, lambda expressions, streams, multithreading, etc. These concepts are the building blocks that will enable you to work on more complex projects and create scalable applications.
1. Object-Oriented Programming (OOP) in Java
As a fully object-oriented language, Java’s OOP principles play a key role in ensuring that well-structured applications can be defined. OOP helps in dividing your code into highly reusable, structured components that are efficient to maintain and extend.
Encapsulation: Encapsulation is the technique of grouping together data (variables) and methods that operate on that data into one entity known as a class. After all, you want to be able to restrict what access the class’s variables have with access modifiers like private, protected, and public and ensure that your data isn’t compromised.
Key OOP Concepts:
Inheritance: inheritance lets you define a class based on an existing class, along with the use of the extends keyword. It encourages code reuse and allows you to leverage existing features.
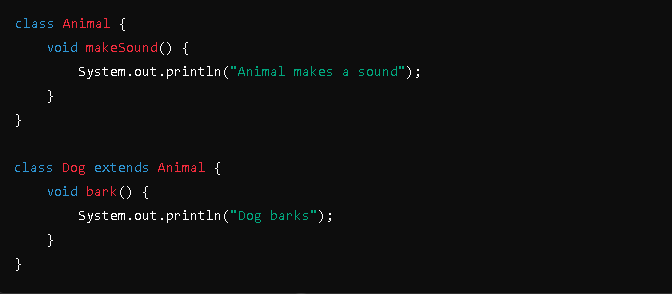
- Polymorphism is the ability to use the same name for different types that are derived from the same base type. It does this through method overloading (different parameters, same method name) and method overriding (a subclass provides an implementation of a method already defined in a parent class).
- Abstraction: The process of hiding complex details and exposing the necessary features. In Java, abstraction is achieved by abstract classes and interfaces.
2. Exception Handling in Java
Error handling is essential to developing reliable software, and Java offers a robust exception-handling mechanism at runtime.
- Error handling is essential to developing reliable software, and Java offers a robust exception-handling mechanism at runtime.
- Checked or Unchecked Exceptions:
- Checked exceptions: It was mandatory to catch checked exceptions (such as IOException) or declare them in the method signature using throws.
- Unchecked exceptions (e.g., NullPointerException) do not have to be explicitly handled.
Exception Handling Syntax:
- Try-Catch Block: This is used to catch exceptions and handle them gracefully.
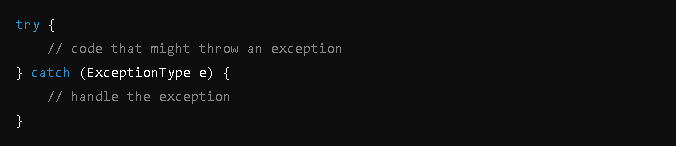
- Finally Block: The finally block is not mandatory but also executed regardless of an exception; hence, it is helpful for cleanup operations like closing files or releasing resources.
- Custom Exceptions: You can define your own exceptions falling under the Exception or RuntimeException classes to manage your application-specific errors.
3. Java Collections Framework
The Java Collections Framework is a collection of classes and interfaces to work with a group of objects. Lists, sets, maps, and more inventory.
- Lists: Lists are ordered collections allowing duplicates. ArrayList and LinkedList are examples of its implementations.

- Sets: Sets are collections with no duplicate elements. These include HashSet and Tree Set.

- Map: Maps are used to store in key-value pairs. Well-known implementations are HashMap and TreeMap.

- Queue: Queues maintain a first in, first out (FIFO) order where the elements are stored. When do you use priority queue? Priority queue is commonly used when you need to prioritize the elements.
Java’s Collections Framework also offers sorting, searching, and other utilities, so it’s a must-have to work with data.
4. Generics in Java
Generics enable you to write flexible and reusable code by letting you define types, which will be specified later when they are used. It provides type safety and helps avoid runtime errors.
Key Concepts of Generics:
- Generic Classes: You can define a class where the type of its members can change using a type parameter.
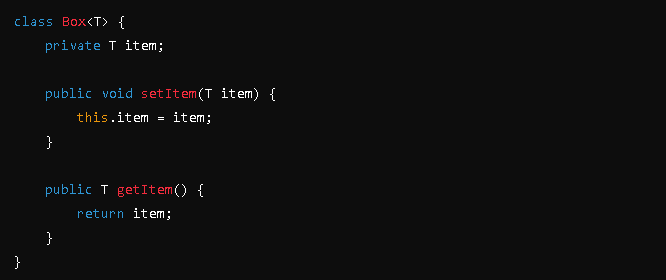
- Bounded Types: The type parameter can be restricted to a specific class or any of its subclasses using extends.

- Wildcard Types: the wildcard (?) enables more flexible input types for methods, which can be handy when receiving types you aren’t necessarily aware of. For example,? T extends allows you to indicate any type that is a subclass of T.
5. Lambda Expressions and Functional Interfaces
Lambda expressions In Java 8, a new construct was introduced called a lambda expression, where you can pass functionality as the argument to the method, therefore making the code cleaner and easier to read.
- Syntax:

Example:

Functional interfaces are interfaces that contain only one abstract method. Examples are Runnable, Callable, and Comparator.
6. Java Streams API
What is the Java 8 Streams API? Java 8 introduced a new abstraction called the Streams API, which helps to work with a sequence of data in a functional way. It supports operations such as filtering, mapping, and reducing data that allow you to process collections in a cleaner and more efficient manner.
Key Stream Operations:
- Filter: Filters out elements based on a condition.
- Map: It transforms every element in stream.
- Reduce: Combines elements into a single representation.
Example:

For large sets of data, streams allow you to write more concise and clearer code.
7. Multithreading in Java
In addition to object-oriented programming, Java also has great support for multithreading, which lets you write programs capable of doing multiple tasks simultaneously. It helps to enhance the performance of applications like servers and real-time systems.
Creating Threads:
- By extending the
Thread
class:
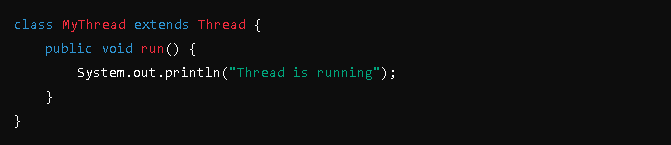
- By implementing the
Runnable
interface:
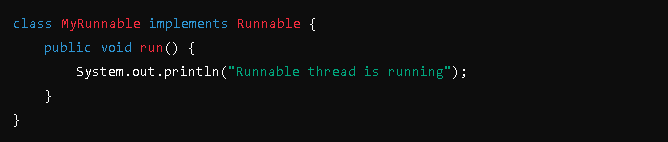
Java’s java.util. and thread pool immediately or schedule with the concurrent package, which provides higher-level concurrency utilities like the ExecutorService.
Conclusion
When you complete these Java intermediate programs, you will become a master, and it will help you to create complex applications. Learning object-oriented principles, exception handling, collections, generics, lambda expressions, streams, and multithreading will improve your code and make you more prepared for programming problems one might encounter in practice.
Practice, and you will become a better Java developer! Check our tutorials and resources to expand your knowledge on these subjects!