Java development can be complex and time-consuming. But, with the right tools, it doesn’t have to be. Using Git for version control, Maven and Gradle for build management, and CI/CD pipelines can make a big difference. This leads to faster and more efficient development cycles, better quality code, and fewer errors.
Git helps manage different versions of code. Maven and Gradle handle build and dependency management well. CI/CD pipelines automate testing, deployment, and monitoring. This means the code is stable and reliable. Developers can then focus on writing code, not managing the process.
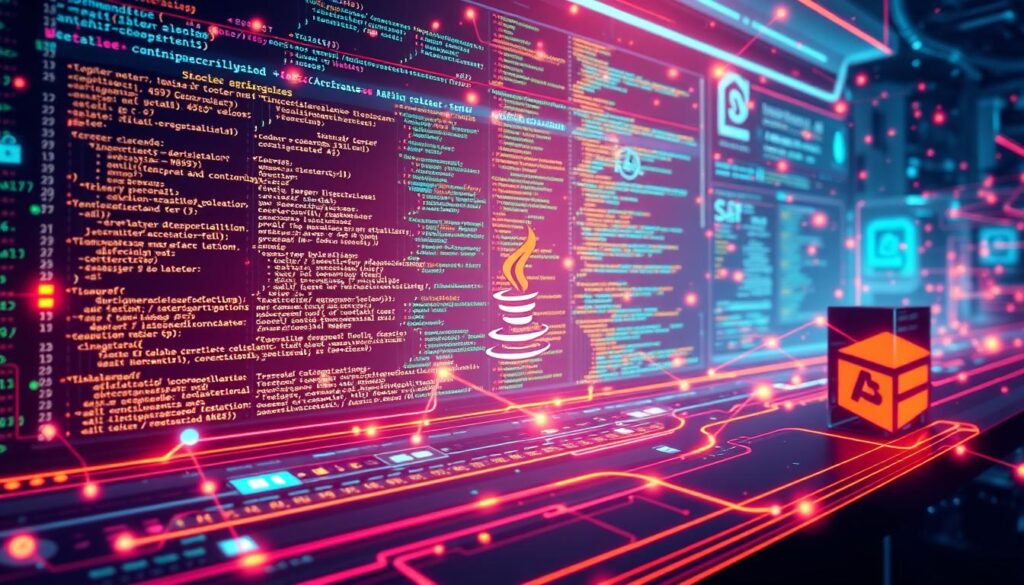
Introduction to Streamlined Java Development
Streamlining java development with Git, Maven/Gradle, and CI/CD boosts productivity and code quality. These tools automate many tasks, freeing developers to concentrate on writing code. This approach improves overall development efficiency and reduces errors.
Key Takeaways
- Java version control with Git helps manage different code versions
- Maven and Gradle provide efficient build management and dependency management
- CI/CD pipelines enable automated testing, deployment, and monitoring
- Streamlined java development improves productivity and code quality
- Git, Maven, Gradle, and CI/CD work together to automate manual tasks
- These tools help reduce errors and improve overall development efficiency
Understanding Modern Java Development Workflows
Modern java development workflows have changed a lot lately. They now focus on being agile, flexible, and efficient. At the core of these workflows are integrated development tools. These tools help developers work better together, reduce mistakes, and make software faster.
Studies show that modern java development uses agile methods, continuous integration, and deployment. This way, developers can work in cycles, get feedback, and adjust quickly. With integrated development tools, they can automate testing and deployment. This lets them spend more time on coding and delivering value.
Some key benefits of java development workflows include:
- Improved collaboration and communication among team members
- Faster time-to-market and reduced cycle times
- Increased quality and reliability of software solutions
- Reduced errors and defects through automated testing and deployment
By understanding and adopting modern java development workflows, developers can get better at their jobs. They can keep up with new trends and technologies. This helps them deliver top-notch software that meets user needs.
Benefits | Description |
---|---|
Improved Collaboration | Enhanced communication and teamwork among developers |
Faster Time-to-Market | Reduced cycle times and accelerated delivery of software solutions |
Increased Quality | Higher quality and reliability of software solutions through automated testing and deployment |
Getting Started with Java Version Control Using Git
Developers use Git for java version control because it’s flexible and scalable. The first step is to create a git repository.
They can make a git repository with the command line or a graphical interface. After creating it, they can add their java code. They use Git commands to commit changes. Git also has features like branching and tagging for managing code versions and working with others.
- Version control: Git helps developers manage code versions, making it easy to track changes and work together.
- Flexibility: Git works well with many development tools and platforms, making it a good choice for java version control.
- Scalability: Git is built to handle big and complex projects, which is why it’s popular for java version control.
By following these steps and using Git for java version control, developers can make their development process smoother. They can also improve teamwork with their team.
Essential Build Management with Maven and Gradle
Build management is key in Java development. Maven and Gradle are top tools for this. They help with automated builds, manage dependencies, and report progress. This makes Java development smoother.
Maven uses POM files for managing projects. Gradle relies on build scripts. Both tools help manage dependencies and automate builds. This makes projects more efficient.
Maven Project Structure and POM Files
Maven’s POM files help organize project structures and dependencies. This makes complex projects easier to handle. Developers can manage their projects well with Maven.
Gradle Build Scripts and Dependencies
Gradle’s build scripts offer flexibility in managing dependencies and builds. This makes it easy to customize the build process. Developers can keep their projects organized and efficient with Gradle.
Choosing Between Maven and Gradle
Choosing between Maven and Gradle depends on project complexity, team size, and build needs. Each tool has its own strengths and weaknesses. Knowing these helps developers pick the right tool for their project.
Implementing Effective CI/CD Pipelines for Java Projects
Creating effective CI/CD pipelines is key for java projects. It makes testing, deployment, and monitoring automated. This catches errors early and speeds up getting the project to market. Tools like Jenkins, Travis CI, or CircleCI help automate these tasks.
Some benefits of CI/CD pipelines for java projects include:
- Improved code quality through automated testing
- Faster deployment and time-to-market
- Enhanced collaboration and visibility among team members
When setting up CI/CD pipelines for java projects, keep these best practices in mind:
- Use a version control system like Git to manage code changes
- Automate testing using tools like JUnit or TestNG
- Use a continuous integration tool like Jenkins to automate the build and deployment process
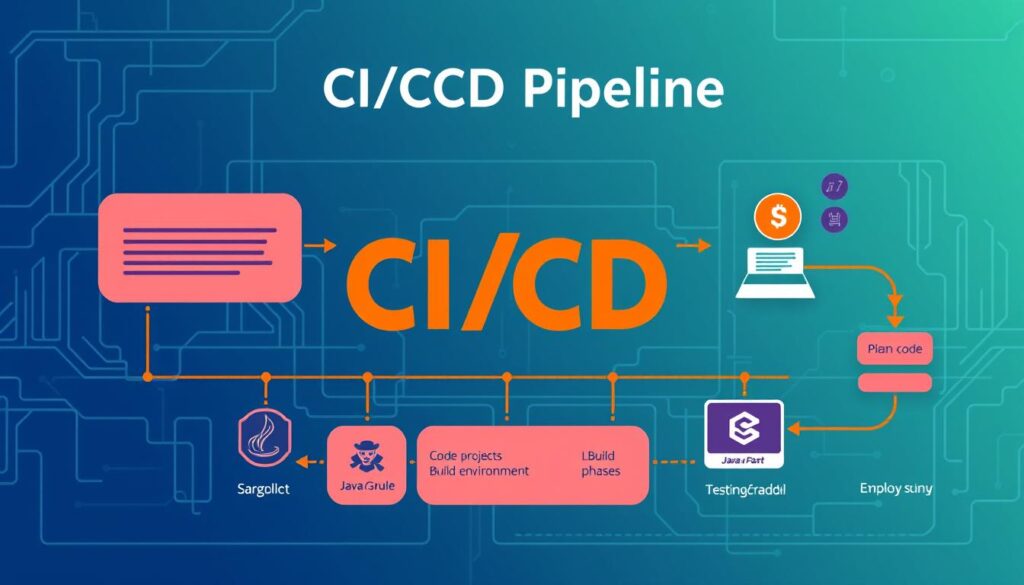
By following these best practices and using the right tools, developers can create effective CI/CD pipelines. This ensures high-quality code and faster time-to-market.
Effective CI/CD pipelines are essential for delivering high-quality java projects quickly and efficiently. By automating testing, deployment, and monitoring, developers can focus on writing code and delivering value to their customers.
Best Practices for Java Code Quality and Testing
Ensuring java code quality is key for reliable and maintainable software. Developers should focus on code review, unit testing, and integration testing. This ensures the code is reliable, easy to maintain, and efficient.
Code review is vital in the development process. It lets developers check code changes against standards and best practices. This step helps find and fix errors, improves code readability, and lowers bug and security risks. Regular code review also boosts team knowledge and collaboration, leading to better code quality.
Code Review Strategies
Effective code review strategies involve a detailed look at the code’s functionality, performance, and security. Developers should use tools like Git for tracking changes and collaborating on reviews. They should also follow guidelines and checklists to ensure the code meets standards.
Unit Testing Frameworks
Unit testing is crucial for java code quality. Frameworks like JUnit and TestNG automate testing of individual code units. This helps developers quickly find and fix errors, ensuring the code is correct and stable.
Integration Testing Approaches
Integration testing is also essential for java code quality. Tools like Mockito and PowerMock automate testing of integrated code. This helps developers identify and fix errors that occur when different components interact, ensuring reliable and efficient code.
By following these best practices, developers can ensure their code is reliable, maintainable, and efficient. Regular code review, unit testing, and integration testing are key to delivering high-quality software that meets standards.
Testing Type | Description |
---|---|
Unit Testing | Testing individual units of code |
Integration Testing | Testing integrated code |
Code Review | Reviewing code changes to ensure quality |
Advanced Debugging Techniques in Java Development
Debugging in Java is key to solving complex problems. Tools like Eclipse, IntelliJ IDEA, or Visual Studio Code help developers. They use these tools to improve their skills and cut down on debugging time.
Advanced techniques include remote debugging and profiling. Remote debugging lets developers fix issues on other machines. Profiling helps find slow parts of the code. These methods are vital for solving tough problems.
Using advanced debugging techniques has many benefits. It boosts skills, cuts down on time spent debugging, and helps fix hard issues. It also makes code run better and be more reliable.
- Improved debugging skills
- Reduced debugging time
- Ability to identify and fix complex issues
- Enhanced code performance and reliability
Developers can use many tools and methods for advanced debugging. For example, they can use the Java Debug Wire Protocol (JDWP) for remote debugging. They can also use tools like Java Mission Control or YourKit for profiling.
By adding advanced debugging to their workflow, Java developers can make their code better. They can work faster and more efficiently. This is crucial in today’s fast-paced world where quick updates are key to success.
Debugging Technique | Description |
---|---|
Remote Debugging | Enables developers to debug code running on remote machines |
Profiling | Enables developers to analyze code performance and identify bottlenecks |
Optimizing Your Development Environment for Maximum Efficiency
To boost developer productivity, it’s key to fine-tune the development environment. This means tweaking the ide configuration to fit your style, like setting up shortcuts and code completion. A top-notch setup can cut down on development time and enhance code quality.
For plugin recommendations, many tools can make coding better. Code analysis and debugging plugins are great for spotting and fixing errors fast. These plugins help developers work more smoothly and write better code.
Key Strategies for Optimization
- Customize your ide configuration to improve coding speed and accuracy
- Utilize plugin recommendations to enhance functionality and productivity
- Implement performance optimization strategies, such as caching and indexing, to improve code performance
Optimizing the development environment boosts productivity and efficiency. This leads to quicker and more efficient development. It also means better software and happier customers.
Common Challenges and Solutions in Java Development Workflows
Java development workflows can be tricky. Issues like managing dependencies, build problems, and code quality are common. To tackle these, developers use tools like Maven and Gradle. They also set up CI/CD pipelines and follow best practices for code and testing.
Some common challenges in java development workflows include:
- Dependency management: managing dependencies between different components of a project
- Build process issues: issues with the build process, such as compilation errors or packaging problems
- Code quality problems: issues with the quality of the code, such as bugs or performance issues
To address these challenges, developers can use solutions like:
- Implementing CI/CD pipelines to automate testing and deployment
- Using build management tools like Maven and Gradle to manage dependencies and build processes
- Following best practices for code quality and testing, such as writing unit tests and conducting code reviews
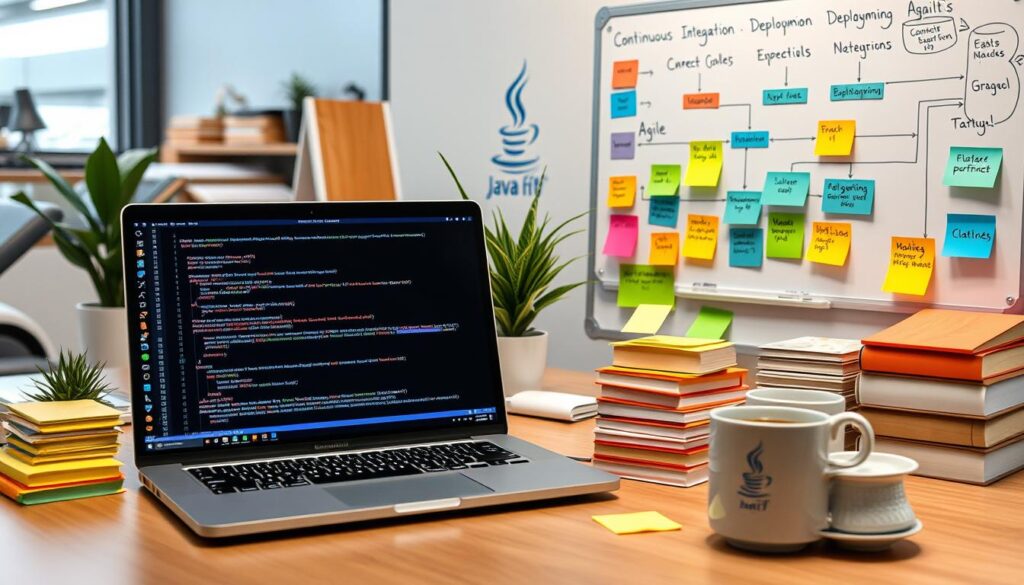
By understanding these common challenges and solutions, developers can improve their skills. They can stay current with java development trends and find effective solutions. This makes their workflows more efficient and effective.
Challenge | Solution |
---|---|
Dependency management | Use build management tools like Maven and Gradle |
Build process issues | Implement CI/CD pipelines to automate testing and deployment |
Code quality problems | Follow best practices for code quality and testing |
Conclusion: Mastering Your Java Development Pipeline
Mastering your Java development pipeline is key to better productivity and efficiency. It involves understanding modern Java workflows, using Git for version control, and setting up CI/CD pipelines. Following best practices for code quality and testing also helps.
When you optimize your development environment, you can work faster and more efficiently. This leads to higher-quality software and happier customers.
To master your Java development pipeline, use tools and techniques that make development smoother. Tools like Git, Maven/Gradle, and CI/CD automate tasks and improve code consistency. They also help teams work together better.
Don’t forget to focus on code quality and testing. This ensures your software meets high standards and gains customer trust.
The journey to mastering your Java development pipeline never ends. New technologies and best practices keep coming. It’s important to stay open to learning and adapt.
By always looking to improve and embracing a growth mindset, you can keep making your development workflow better. This leads to outstanding results for your team and organization.
FAQ
What are the key components of modern Java development workflows?
Modern Java development workflows include Git for version control. They also use Maven or Gradle for build management. Plus, CI/CD pipelines for automated testing and deployment.
How can Git help streamline Java development?
Git helps manage different code versions and collaborate with others. It keeps a clear history of code changes. This ensures the stability and reliability of Java projects.
What are the benefits of using build management tools like Maven and Gradle?
Maven and Gradle manage dependencies and automate builds. They offer reporting features too. These tools simplify Java project management, improve collaboration, and ensure consistent quality.
How can CI/CD pipelines improve Java development workflows?
CI/CD pipelines automate testing, deployment, and monitoring. They help catch errors early and reduce time-to-market. This ensures code reliability and quality.
What are some best practices for ensuring Java code quality and testing?
Best practices include code reviews and using JUnit and TestNG for testing. Integration testing with Mockito and PowerMock is also key. These practices make code reliable and maintainable.
What are some advanced debugging techniques that can help in Java development?
Advanced techniques include remote debugging and profiling. They help identify and fix complex issues by analyzing code performance and behavior.
How can developers optimize their development environment for maximum efficiency?
Developers can optimize their environment by customizing IDEs and using plugins. Implementing performance strategies also helps. This improves coding speed and accuracy.
What are some common challenges in Java development workflows and how can they be addressed?
Challenges include dependency management and build process issues. Code quality problems are common too. Using build tools, CI/CD pipelines, and best practices can solve these issues.