Java is a popular and versatile programming language well-suited for developing scalable, maintainable, and high-performance applications. The applications tend to get complex, and it becomes essential for developers to know advanced Java concepts so they can work even smarter to optimise their applications and write effective code. This guide includes key topics like JVM internals, performance tuning, design patterns, the principle of reflection, database connectivity, Java module systems, etc.
1. JVM Internals: How the Java Virtual Machine Works
The Java Virtual Machine (JVM) is at the heart of Java’s “write once, run anywhere” philosophy. The hardware and operating system are abstracted away by the JVM, allowing Java applications to be executed on any device or operating system.
Key components of the JVM:
- Class Loader: Class Loader helps in loading the classes in Java into memory. It is responsible for loading classes, linking classes, and initializing classes during runtime.
- Memory Management:
- Heap Memory: The heap is part of memory where all the Java objects get stored during the runtime. The heap is globally shared across all threads.
- Stack Memory: Every thread in Java has its own stack, which is used to hold method calls, local variables, and references to objects.
- Method Area: It contains the class information like class structure, fields, method, and constant pools.
- PC Register (Program Counter): It holds the address of the next instruction to be executed.
- Native Method Stack: Stores data for native code like C, C++, etc.
Garbage Collection in JVM:
Java has Garbage Collection (GC) to automatically reclaim memory that is no longer being used. GC algorithms include:
- Serial GC: It is a single-threaded garbage collector for small applications.
- Parallel GC: Multi-threaded GC for increased throughput.
- G1 GC (Garbage First): Focuses on reducing pause times; suitable for large applications with real-time constraints.
2. JVM Performance Tuning: Optimizing Java Applications
To make Java applications perform at their best, you need to optimize the JVM for your application needs. Tuning JVMs for performance is about managing memory allocation, choosing the optimal garbage collection method, and keeping an eye on how the application acts and reacts.
Heap Sizing:
Setting the big index Info_MAX_MEMORY in the options of INFO()
can also help to reduce memory usage. To optimize heap memory usage, always set the JVM flags -Xms (initial heap size) and -Xmx (maximum heap size).

Garbage Collection Tuning:
Choosing the Garbage Collection algorithm is critical to improving the performance:
- G1 Garbage Collector: A low-latency GC algorithm that works by dividing the heap into regions.
- Parallel GC: For applications with high throughput.
Monitoring Tools:
- JVisualVM: Shows real-time detail on heap usage, thread activity and garbage collection.
- JConsole: Monitors CPU usage, memory consumption, and garbage collection

3. Design Patterns in Java: Best Practices for Code Reusability
Design patterns are established solutions to common software design problems. The code can be more maintainable, scalable, and reusable, which Java developers often do by following design patterns.
Popular Design Patterns in Java:
- Singleton Pattern: Guarantees a class has one and only instance. which is typically used by logging, configuration settings, and connection management.
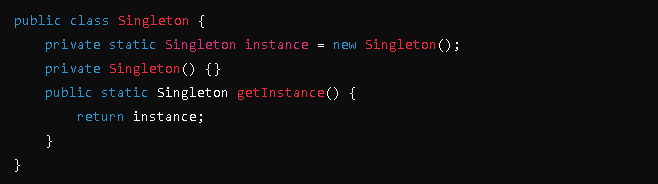
- Factory Pattern: Only use it to create objects without specifying the class. This design pattern helps to achieve loose coupling of client with class being instantiated.
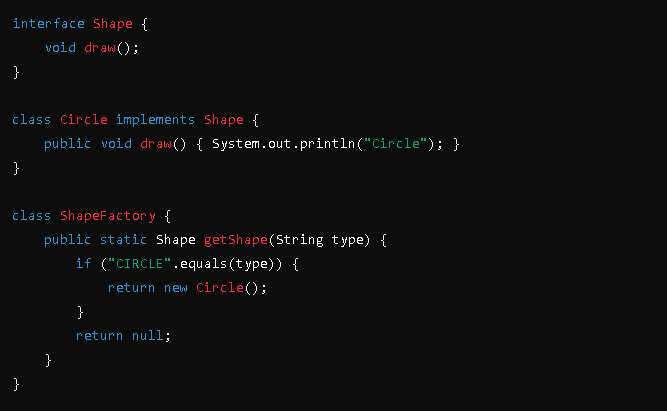
- Strategy Pattern: Encapsulates various algorithms and makes them interchangeable. No more complex conditional logic is required for that.
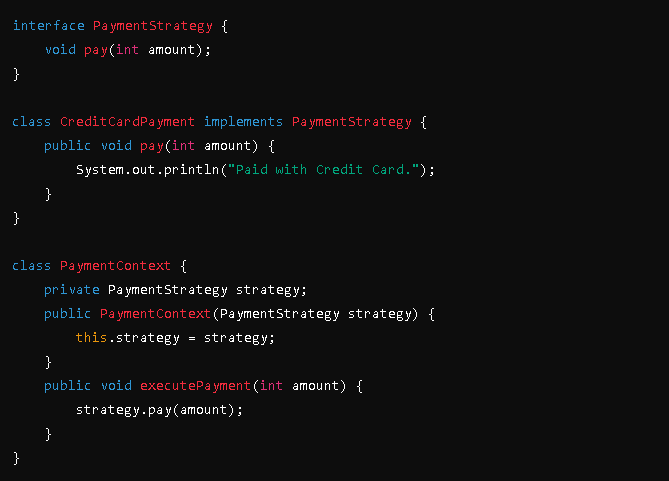
- Observer Pattern: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
4. Java Reflection API: Dynamic Class and Object Manipulation
The Java Reflection API is used to inspect and manipulate Java classes and objects at runtime. Frameworks like Spring and Hibernate heavily use reflection to dynamically invoke methods, instantiate objects, and discover classes.
Key Features of Reflection:
- Class Discovery: Retrieve information from classes dynamically during runtime (methods, fields, etc.).

- Dynamic Method Invocation: Enables the runtime invocation of methods without prior knowledge of their signatures at compile time, providing higher flexibility for frameworks and libraries.
- Creating Instances Dynamically: Create new instances of classes at runtime using reflection.

5. JDBC and Database Connectivity: Working with Relational Databases
JDBC (Java Database Connectivity) is the standard API to connect Java applications with all relational databases such as MySQL, PostgreSQL, Oracle, etc. JDBC is a set of methods that let you query or update databases from Java applications.
Key JDBC Components:
- DriverManager: Monitors the drivers for the databases and connects the application to the database.
- Connection: Allow for connecting the data to the database.
- Statement/PreparedStatement: Used for executing your SQL queries and updates.
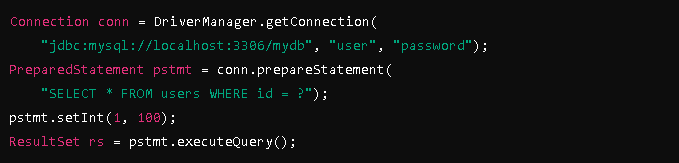
Best Practices:
- PreparedStatements: Also used for securing against SQL injection attacks and for enhancing coding performance by precompiling SQL queries.

- Connection Pooling: Queues connections for reusing them rather than creating new ones for every request. They can use efficient connection pooling libraries like HikariCP and Apache DBCP.
6. Java Modules (JPMS): A Guide to Modularity in Java
The Java Platform Module System (JPMS) was introduced in Java 9, and it enables developers to create applications with better maintenance and security characteristics. JPMS allows you to further modularize big applications into small, reusable packages, therefore making them more scalable and improving memory overhead.
Modular Design:
JPMS allows applications to declare dependencies on other modules and control which of its packages are visible to external modules. The module-info. The module dependencies are declared in the module-info.

Benefits of Modularity:
- Strong Encapsulation: By default, only explicitly exported packages are available to other modules.
- Enhanced Maintainability: With the slicing of bulky applications into modules, managing them becomes a lot simpler.
- Lesser Memory Footprint: Only those components of the JDK that are required are loaded, leading to quicker startup and lower memory utilization.
Conclusion
Advanced-level Java topics are critical concepts to master for developers needing to architect high-performance, scalable, and maintainable applications. With the right knowledge of the JVM workings and performance improvements, the implementation of required design patterns, experience in reflection, database interactions, and modular application development, Java developers could be experts!